 |
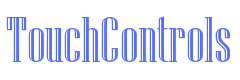 |
iOS Framework (for XCode Swift & Objective C)
|
|
|
The Rustemsoft's UIDataGrid View XCode control seems a little better than the standard UITableView control. It provides a flexible and forceful way to represent a data source. You can extend the UIDataGrid View control in a number of ways to build custom behaviors into your iPad/iPhone apps. While you may design your own types of cells the XCode UIDataGrid View control is mostly column-based, rather than cell-based. As a result, to attain most tasks, you have to work with the columns, not the cells themselves.
Beginning February 1, 2020 TouchControls iOS Framework includes 64-bit support, built with the iOS 8 SDK and Xcode 6.
|
|
TouchControls iOS Framework library from Rustemsoft LLC is Swift & Objective C software package specifically designed for Xcode developers. The library allows you to use all strengths of the Rustemsoft UIDataGrid control without waiving the user interface elements your customers need.
The UIDataGrid control provides a powerful and flexible way to display data in a tabular format. You can use the UIDataGrid control to show read-only views of a small amount of data, or you can scale it to show editable views of very large sets of data.
|
|
|

You can extend the UIDataGrid control in a number of ways to build custom behaviors into your iOS apps. For example, you can programmatically specify your own set of displayed columns, and you can create your own types of cells. You can easily customize the appearance of the UIDataGrid control by choosing among several properties.
|
|
|
|

Several types of data stores (JSON, plist, XML, and a mutable array object) can be used as a data source. The UIDataGrid control provides a user interface to this data sources, displays the tabular data in a scrollable grid, and allows for updates to the data source. In cases where the UIDataGrid is bound to a data source with a single table the data appears in simple rows and columns, as in a spreadsheet. The UIDataGrid control is one of the most useful and flexible controls for Xcode iOS development. As soon as the UIDataGrid control is set to a valid data source, the control is automatically populated, by creating columns and rows based on the columns objects structure you defined in your project's code.

By using the TouchControls Framework you can create your own set of DataGrid Column objects that defines custom column for the iOS UIDataGrid control and add them to the Grid Columns collection. A column is an object that defines what the column looks and behaves like, including such things as color, font, and the presence of controls that will handle linked field in a data source with the use of a UIPickerView Control, a Text Field view, a DateTime format Picker and other UIView controls.

Also we have built Xcode sample projects that describe the concepts and techniques that you can use to build UIDataGrid features into your apps.
Rustemsoft TouchControls Framework library contains the following DataGrid Columns:
|
|
|
|